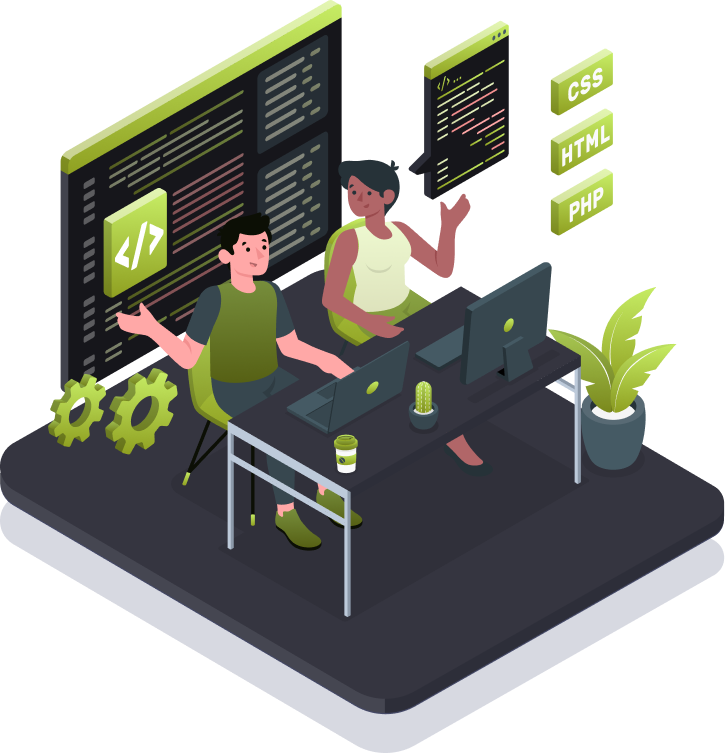
What Is PHP IP Geolocation Software?
PHP IP geolocation software identifies a visitor’s location based on their IP address using PHP code. With PHP IP geolocation service, developers can program HTML or dynamic location-based websites without any hassle.
When you get user geolocation using PHP, you’ll have enough data to geo-localize different aspects of your webpage for each visitor.
Simply integrate our geolocation PHP script, and you’re good to go.
Features
Features of the PHP Geolocation Plugin
The PHP IP Geolocation plugin offers comprehensive data with every visitor's IP. If you don’t need all of that data, you can also filter for specific variables.
Get City From IP Using PHP
Have a special offer for specific cities? Use the PHP API to get a city from IPs and make city-specific customizations to your pages in a snap. Using geoPlugin, you can also get country by IP PHP API.
Get Country by IP Using PHP
Send a request, get country from IP address using PHP API and show customers what’s available in their country.
PHP Geolocation Currency Converter
Get geolocation from IPs using PHP and use the exchange rate to automatically display prices in each visitor’s local currency. We support a wide range of global currencies for maximum convenience.
How Does the GeoPlugin PHP Web Service Work?
The GeoPlugin PHP GPS location web service retrieves visitor location data in seconds using their IP address. You can access the country, city, zip code, time zone, latitude, longitude, currency, language, and more using client’s IP address.
The GeoPlugin PHP Web Service retrieves geolocation data by cross-referencing the visitor’s IP address with a global IP database. This database matches IPs to locations and calculates location details like country and city for accurate geotargeting.
Simply embed our PHP IP plugin script, and the moment visitors land on your site, their data will be at your fingertips.
Example of a PHP Geolocation Script
The following is a PHP geolocation example request for an IP’s geolocation:
echo( var_export((unserialize(file_get_contents('http://www.geoplugin.net/php.gp?ip='. $_SERVER['REMOTE_ADDR'])));
array ( 'geoplugin_request' => 'xx.xx.xx.xx', (IP) 'geoplugin_status' => 200, 'geoplugin_delay' => '1ms', 'geoplugin_credit' => 'Some of the returned data includes GeoLite2 data created by MaxMind, available from https://www.maxmind.com.', 'geoplugin_city' => 'Karachi', 'geoplugin_region' => 'Sindh', 'geoplugin_countryName' => 'Pakistan', 'geoplugin_latitude' => '24.9239', 'geoplugin_longitude' => '67.1423', 'geoplugin_currencyCode' => 'PKR', 'geoplugin_currencySymbol' => '₨', 'geoplugin_currencyConverter' => '277.5742', )
PHP Geolocation Class
Our laravel or PHP IP API comes with complete setup instructions. Download the geoPlugin PHP class for a detailed example and tips to guide you through setup and integration.
The same geolocation web services are available via JavaScript, ASP XML, SSL, and JSON, too.
Have questions? Get in touch!